What is std::string C++?
C++ has in its definition a way to represent a sequence of characters as an object of the class. This class is called std:: string. String class stores the characters as a sequence of bytes with the functionality of allowing access to the single-byte character.
Table of Contents
What is string :: NPOS?
What is string::npos: It is a constant static member value with the highest possible value for an element of type size_t. It actually means until the end of the string. It is used as the value for a length parameter in the string’s member functions. As a return value, it is usually used to indicate no matches.

How do I find a word in a sentence C++?
Dry run
- Run a for loop, starting from 0 to the size of the string – 1, i.e., from i=0 to i==str. size() .
- Check if str[i] is a space character.
- The for loop will run to the end of the string, and the number of spaces will be counted.
- Increment the number of spaces by 1 to get the number of words.
Can you index strings in C++?
Indexing lets you use a numeric indicator to directly access individual characters in a string. String indexing C++ uses a zero-based technique to index strings: the first character has index 0, the next has index 1, and so on. You’ll learn string indexing syntax and practice with various examples in this article.
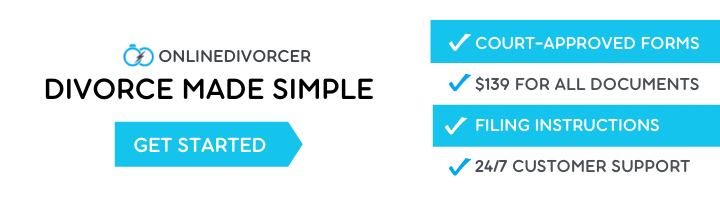
How do I print a STD variable string?
Print a String in C++
- Use std::cout and << Operator to Print a String.
- Use std::copy Algorithm to Print a String.
- Use printf() Function to Print a String.
Is std::string a pointer?
std::string::data Returns a pointer to an array that contains the same sequence of characters as the characters that make up the value of the string object.
How do you find a string in a sentence?
If you have the string: string sample = “If you know what’s good for you, you’ll shut the door!”; And you want to find where it is in a sentence, you can use the IndexOf method. A non -1 answer means the string has been located.
What library is std::string in?
the C++ standard library
The string class is part of the C++ standard library.
What is std::string data?
std::string::data() in C++ The data() function writes the characters of the string into an array. It returns a pointer to the array, obtained from conversion of string to the array. Its Return type is not a valid C-string as no ‘\0’ character gets appended at the end of array.
How do you find the first occurrence of a string?
String find is used to find the first occurrence of sub-string in the specified string being called upon. It returns the index of the first occurrence of the substring in the string from given starting position. The default value of starting position is 0. Function Template: size_t find (const string& str, size_t pos = 0);
How to search for a specific sub-string in a string?
s : The sub-string to be searched, given as C style string. pos : The initial position from where the string search is to begin. The function returns the index of the first occurrence of sub-string, if the sub-string is not found it returns string::npos (string::pos is static member with value as the highest possible for the size_t data structure).
How do I search a string stream?
The only effective way to search a string stream is to convert it to a std::string, first.
What is strstr and POS in C?
str : The sub-string to be searched. s : The sub-string to be searched, given as C style string. pos : The initial position from where the string search is to begin.